Developer
Manager
Designer
User
Integrate within 30 minutes
Video Collaboration
Video Collaboration
with
with
Sync Players
Sync Players

Frame.io-style
Synced Players

Frame.io-style
Synced Players


Richard
München, Germany


Eva Ash
Miami, USA
Trusted by
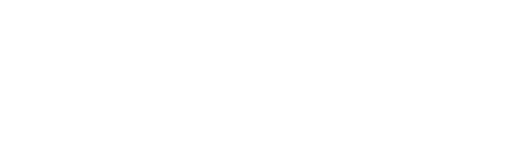



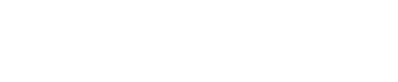





Trusted by
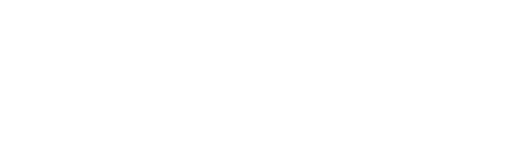



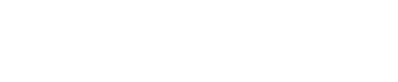





Trusted by
Trusted by
Connected to scalable realtime backend infrastructure.
Embed fully customizable powerful experiences with a few lines of code.
Embed fully customizable powerful experiences with a few lines of code.
Embed fully customizable powerful experiences with a few lines of code.
Code Snippet
import { useEffect } from "react";
import { useVeltClient } from '@veltdev/react';
import { generateUserData } from './util/user';
import styles from './App.module.css';
import Layout from './components/layout/Layout';
import Breadcrumbs from './components/breadcrumbs/Breadcrumbs';
import Explorer from './components/explorer/Explorer';
import Preview from './components/preview/Preview';
import SplitPane from './components/split-pane/SplitPane';
import Timeline from "./components/timeline/Timeline";
import Controls from "./components/controls/Controls";
import FileExplorer from "./components/file-explorer/FileExplorer";
import Stage from "./components/stage/Stage";
import { VeltPresence } from "@veltdev/react";
const App = () => {
/**
* Velt Code Example
* Initializes the Velt SDK.
*/
const { client } = useVeltClient();
useEffect(() => {
if (!client) return;
const user = generateUserData();
client.identify(user);
client.setDocumentId('video_project_id');
}, [client]);
useEffect(() => {
if (!client) return;
client.getPresenceElement().getOnlineUsersOnCurrentDocument().subscribe((users: any) => {
if (users === null) return;
if (users.length === 0) {
const isDataReset = window.sessionStorage.getItem('_snippyly_demo_reset');
if (isDataReset === null) {
console.log('reset data!!');
fetch(
"https://us-central1-snippyly-sdk-prod.cloudfunctions.net/resetDemoData",
{
headers: { "Content-Type": "application/json" },
method: "POST",
body: JSON.stringify({ documentId: 'video_project_id' }),
}
);
window.sessionStorage.setItem('_snippyly_demo_reset', 'true');
}
}
});
}, [client]);
return (
<div className={styles['app-container']}>
<Layout
topbar={
<div className={styles['topbar']}>
<Breadcrumbs />
{/**
* Velt Code Example
* Feature: Presence
*/}
<div className={styles['presence-container']}>
<VeltPresence />
</div>
</div>
}
mainWindow={
<SplitPane
left={<Explorer />}
right={<Preview />}
/>
}
bottomPane={
<div className={styles['bottom-container']}>
<div className={styles['control-bar']}>
<Controls />
<Timeline />
</div>
<div className={styles['interact-bar']}>
<FileExplorer />
<Stage />
</div>
</div>
}
/>
</div>
);
};
export default App;
Code Snippet
import { useEffect } from "react";
import { useVeltClient } from '@veltdev/react';
import { generateUserData } from './util/user';
import styles from './App.module.css';
import Layout from './components/layout/Layout';
import Breadcrumbs from './components/breadcrumbs/Breadcrumbs';
import Explorer from './components/explorer/Explorer';
import Preview from './components/preview/Preview';
import SplitPane from './components/split-pane/SplitPane';
import Timeline from "./components/timeline/Timeline";
import Controls from "./components/controls/Controls";
import FileExplorer from "./components/file-explorer/FileExplorer";
import Stage from "./components/stage/Stage";
import { VeltPresence } from "@veltdev/react";
const App = () => {
/**
* Velt Code Example
* Initializes the Velt SDK.
*/
const { client } = useVeltClient();
useEffect(() => {
if (!client) return;
const user = generateUserData();
client.identify(user);
client.setDocumentId('video_project_id');
}, [client]);
useEffect(() => {
if (!client) return;
client.getPresenceElement().getOnlineUsersOnCurrentDocument().subscribe((users: any) => {
if (users === null) return;
if (users.length === 0) {
const isDataReset = window.sessionStorage.getItem('_snippyly_demo_reset');
if (isDataReset === null) {
console.log('reset data!!');
fetch(
"https://us-central1-snippyly-sdk-prod.cloudfunctions.net/resetDemoData",
{
headers: { "Content-Type": "application/json" },
method: "POST",
body: JSON.stringify({ documentId: 'video_project_id' }),
}
);
window.sessionStorage.setItem('_snippyly_demo_reset', 'true');
}
}
});
}, [client]);
return (
<div className={styles['app-container']}>
<Layout
topbar={
<div className={styles['topbar']}>
<Breadcrumbs />
{/**
* Velt Code Example
* Feature: Presence
*/}
<div className={styles['presence-container']}>
<VeltPresence />
</div>
</div>
}
mainWindow={
<SplitPane
left={<Explorer />}
right={<Preview />}
/>
}
bottomPane={
<div className={styles['bottom-container']}>
<div className={styles['control-bar']}>
<Controls />
<Timeline />
</div>
<div className={styles['interact-bar']}>
<FileExplorer />
<Stage />
</div>
</div>
}
/>
</div>
);
};
export default App;
Code Snippet
import { useEffect } from "react";
import { useVeltClient } from '@veltdev/react';
import { generateUserData } from './util/user';
import styles from './App.module.css';
import Layout from './components/layout/Layout';
import Breadcrumbs from './components/breadcrumbs/Breadcrumbs';
import Explorer from './components/explorer/Explorer';
import Preview from './components/preview/Preview';
import SplitPane from './components/split-pane/SplitPane';
import Timeline from "./components/timeline/Timeline";
import Controls from "./components/controls/Controls";
import FileExplorer from "./components/file-explorer/FileExplorer";
import Stage from "./components/stage/Stage";
import { VeltPresence } from "@veltdev/react";
const App = () => {
/**
* Velt Code Example
* Initializes the Velt SDK.
*/
const { client } = useVeltClient();
useEffect(() => {
if (!client) return;
const user = generateUserData();
client.identify(user);
client.setDocumentId('video_project_id');
}, [client]);
useEffect(() => {
if (!client) return;
client.getPresenceElement().getOnlineUsersOnCurrentDocument().subscribe((users: any) => {
if (users === null) return;
if (users.length === 0) {
const isDataReset = window.sessionStorage.getItem('_snippyly_demo_reset');
if (isDataReset === null) {
console.log('reset data!!');
fetch(
"https://us-central1-snippyly-sdk-prod.cloudfunctions.net/resetDemoData",
{
headers: { "Content-Type": "application/json" },
method: "POST",
body: JSON.stringify({ documentId: 'video_project_id' }),
}
);
window.sessionStorage.setItem('_snippyly_demo_reset', 'true');
}
}
});
}, [client]);
return (
<div className={styles['app-container']}>
<Layout
topbar={
<div className={styles['topbar']}>
<Breadcrumbs />
{/**
* Velt Code Example
* Feature: Presence
*/}
<div className={styles['presence-container']}>
<VeltPresence />
</div>
</div>
}
mainWindow={
<SplitPane
left={<Explorer />}
right={<Preview />}
/>
}
bottomPane={
<div className={styles['bottom-container']}>
<div className={styles['control-bar']}>
<Controls />
<Timeline />
</div>
<div className={styles['interact-bar']}>
<FileExplorer />
<Stage />
</div>
</div>
}
/>
</div>
);
};
export default App;
Playback
Playback
Playback
•
•
•
Editing
Editing
Editing
•
•
•
Analyzing
Analyzing
Analyzing
Playback
Playback
Playback
Playback
Editing
Editing
Editing
Editing
Analyzing
Analyzing
Analyzing
Analyzing
Playback
Playback
Playback
•
•
•
Editing
Editing
Editing
•
•
•
Analyzing
Analyzing
Analyzing
Playback
Watch Together
Watch Together
Sync your video player with others in the same document for a shared and immersive viewing experience

Video Players
view all use cases



1



Editing
Coordinated Understanding
Coordinated Understanding
Ensure everyone is on the same page by synchronizing video playback, fostering shared insights and discussions

Video Editors
view all use cases
2
Analyzing
Analyze moments together
Analyze moments together
Point out iterations frame by frame together on the same frame

Session Replay Tool
view all use cases



3
Increase engagement by
10X

“We were able to add it into our codebase within 10 minutes and were live in next 20”
Brendon Small
CPO @Typeform

“We were able to add it into our codebase within 10 minutes and were live in next 20”
Brendon Small
CPO @Typeform


Integrates deeply within your tech stack.
Integrates deeply within your tech stack.
Integrates deeply within your tech stack.
Works across all major web frameworks.
Works across all major web frameworks.
Works across all major web frameworks.

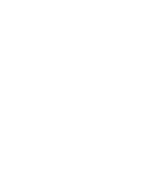
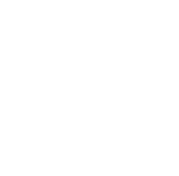


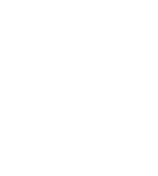

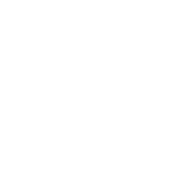
Frequently Asked Questions
Frequently Asked Questions

How long does it take to integrate with Velt SDK?

How long does it take to integrate with Velt SDK?

Which frameworks do you support?

Which frameworks do you support?

Do you offer any volume discounts?

Do you offer any volume discounts?

Do you offer any discounts for startups?

Do you offer any discounts for startups?

How secure is Velt SDK?

How secure is Velt SDK?

How reliable and scalable is Velt SDK?

How reliable and scalable is Velt SDK?
Use Cases